일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
- 백준
- pwnable.kr
- Deadlock
- BFS
- samsung research
- 삼성리서치
- dfs
- fork
- 프로세스
- Brute Force
- 알고리즘
- 데드락
- segmentation
- 삼성기출
- paging
- BOJ
- 스케줄링
- 시뮬레이션
- 운영체제
- ascii_easy
- 완전탐색
- 가상메모리
- exec
- 컴공복전
- higunnew
- 동기화문제
- Memory Management
- 백트래킹
- 구현
- 김건우
- Today
- Total
gunnew의 잡설
BOJ_17825_주사위윷놀이 [DFS by Back Tracking] 본문
PS 백준 소스 코드 모음 : https://github.com/kgw4073/Problem-Solving
https://www.acmicpc.net/problem/17825
17825번: 주사위 윷놀이
첫째 줄에 주사위에서 나올 수 10개가 순서대로 주어진다.
www.acmicpc.net
이 문제를 처음 풀기 시작했을 때 한 20분 동안은 멍했다. 분명히 완전 탐색인데 어떻게 '구현'하지? 뭔가 문제가 상당히 난잡해 보였다. 그도 그럴 것이 밑에 제시된 그림만 봐도 현기증 나게 생겼다. 이건 뭐 어쩌라는 거지... 20분은 그렇게 날렸다.
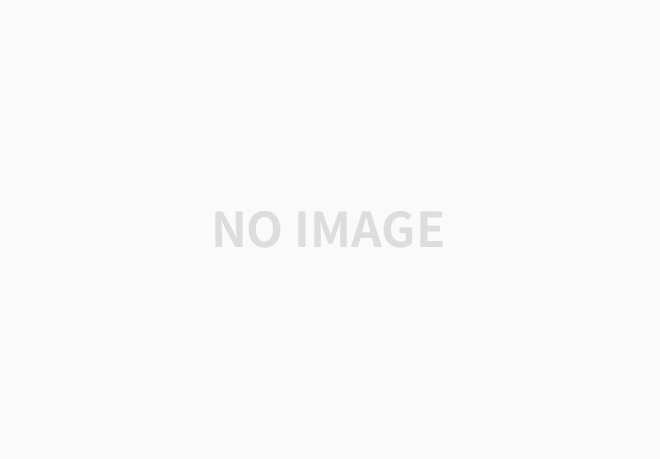
그러다가 실제 시험이라고 생각하니 정신이 들었다. 기존 문제처럼 정갈하게 연결 상태가 주어진 것도 아니고, 친절하게 그림으로 설명을 첨부해 주지도 않았다. 그러면 할 수 있는 것은, 어차피 저 위의 윷놀이 판은 고정되어 있으니 직접 연결하는 수밖에... 바로 다음과 같이 임의로 번호를 매겨 연결해 버렸다. 그리고 그 번호에 해당하는 점수를 저장하는 배열도 만들었다.
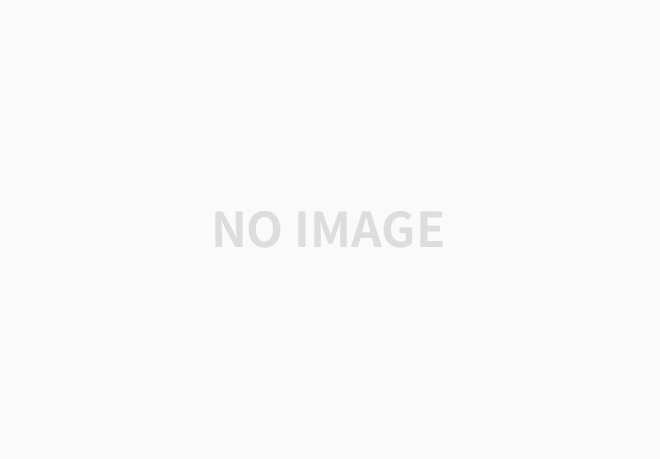
일단 이렇게 연결하고 나면 일반적인 back tracking과 동일하다. 조건 문만 적절히 넣어주면 끝난다. 코드에 주석을 달아 놓았으니 코드를 보자.
혹시 이 다른 코드들도 보고 싶으면
PS 백준 소스 코드 모음 : https://github.com/kgw4073/Problem-Solving
kgw4073/Problem-Solving
Algorithm Problem Solution. Contribute to kgw4073/Problem-Solving development by creating an account on GitHub.
github.com
이것도 보자.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
|
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
const int endPoint = 21;
// 32개의 노드들을 그래프로 연결
vector<int> graph[33];
// score는 32개의 노드들 위에 쓰여있는 점수
int score[33];
// state는 해당 인덱스 노드 위에 말이 있는지 없는지
int state[33];
// 0 ~ 3번 말이 현재 어느 위치에 있는지
int markerNode[4];
// 주사위 결과
int dice[10];
// 0 ~ 3번 말들이 끝났는지 안끝났는지
bool finished[4] = { false, };
// 답
int answer = 0;
void solve(int depth, int total) {
if (depth == 10) {
answer = max(answer, total);
return;
}
// 0 ~ 3번 말 탐색
for (int i = 0; i < 4; i++) {
// 도착 점에 있는 말이면 탐색 안해
if (finished[i]) {
continue;
}
int currentNode = markerNode[i];
int nextNode;
// 현재 노드가 5, 10, 15이면 파란 선 따라 감
if (currentNode == 5 || currentNode == 10 || currentNode == 15) {
int count = dice[depth] - 1;
nextNode = graph[currentNode][1];
while (count--) {
nextNode = graph[nextNode][0];
}
}
else {
int count = dice[depth];
nextNode = markerNode[i];
while (count--) {
nextNode = graph[nextNode][0];
}
}
// 다음 노드가 끝점이어도 일단 탐색하고 다음 말로 넘어감
if (nextNode == endPoint) {
state[currentNode] = 0;
state[nextNode] = 1;
markerNode[i] = nextNode;
finished[i] = true;
solve(depth + 1, total + score[nextNode]);
finished[i] = false;
state[nextNode] = 0;
state[currentNode] = 1;
markerNode[i] = currentNode;
continue;
}
// 다음 노드에 뭐 있으면 탐색 안함
if (state[nextNode]) {
continue;
}
// 다음 노드에 아무 말도 없고 끝점도 아니어도 탐색
markerNode[i] = nextNode;
state[currentNode] = 0;
state[nextNode] = 1;
solve(depth + 1, total + score[nextNode]);
state[nextNode] = 0;
state[currentNode] = 1;
markerNode[i] = currentNode;
}
}
int main() {
// 이 밑에 코드는 직접 그래프 번호 매겨서 연결
for (int i = 0; i <= 20; i++) {
graph[i].emplace_back(i + 1);
score[i] = i * 2;
}
graph[5].emplace_back(22);
score[22] = 13;
graph[22].emplace_back(23);
score[23] = 16;
graph[23].emplace_back(24);
score[24] = 19;
graph[24].emplace_back(25);
score[25] = 25;
graph[25].emplace_back(26);
score[26] = 30;
graph[26].emplace_back(27);
score[27] = 35;
graph[27].emplace_back(20);
graph[10].emplace_back(28);
score[28] = 22;
graph[28].emplace_back(29);
score[29] = 24;
graph[29].emplace_back(25);
graph[15].emplace_back(30);
score[30] = 28;
graph[30].emplace_back(31);
score[31] = 27;
graph[31].emplace_back(32);
score[32] = 26;
graph[32].emplace_back(25);
graph[21].emplace_back(21);
for (int i = 0; i < 10; i++) {
cin >> dice[i];
}
solve(0, 0);
cout << answer;
}
|
cs |
'Algorithm' 카테고리의 다른 글
BOJ_17472_다리만들기2 [Brute forcing by DFS] (0) | 2020.02.06 |
---|---|
BOJ_5373_큐빙 [Brute Brute Brute Forcing, Simulation] (0) | 2020.01.26 |
BOJ_15684_사다리조작(C++) [DFS Back tracking] (0) | 2020.01.23 |
BOJ_12100_2048(C++) [Brute forcing by DFS] (0) | 2020.01.21 |
BOJ_13460_구슬탈출(C++) [BFS] (0) | 2020.01.19 |